반응형
C#에서 MSSQL과 연동해서 데이터조회 및 삽입하는 방법에 대해 알아보겠습니다.
1. 테스트 DB생성
아래와 스크립트문을 이용해 sampledb라는 데이터베이스에 UserInfo테이블을 생성합니다.
1
2
3
4
5
6
7
8
9
10
11
|
create database sampledb
go
use sampledb
go
create table [UserInfo]
(
id int,
name varchar(30)
)
|
2. MSSQL연결하는 MssqlLib.cs클래스 파일생성
MSSQL데이터베이스와 연결하기 위해서는 System.Data.SqlClient 네임스페이스를 이용합니다. SQL서버에 연결하기위해서는 SqlConnection개체를 이용하고, SqlCommand ,SqlDataAdapter를 이용해서 데이터를 저장,조회처리합니다.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data.SqlClient;
using System.Data;
namespace MssqlTestProject
{
class MssqlLib
{
// 접속테스트
public bool ConnectionTest()
{
string connectString = string.Format("Server={0};Database={1};Uid ={2};Pwd={3};", "127.0.0.1",
"sampledb", "test", "1234!");
try
{
using (SqlConnection conn = new SqlConnection(connectString))
{
conn.Open();
}
return true;
}
catch (Exception)
{
return false;
}
}
//데이터조회
public void SelectDB()
{
string connectString = string.Format("Server={0};Database={1};Uid ={2};Pwd={3};", "127.0.0.1",
"sampledb", "test", "1234!");
string sql = "select * from UserInfo";
using (SqlConnection conn = new SqlConnection(connectString))
{
conn.Open();
SqlCommand cmd = new SqlCommand(sql, conn);
SqlDataReader dr = cmd.ExecuteReader();
dr.Close();
}
}
//INSERT처리
public void InsertDB(int id, string name)
{
string connectString = string.Format("Server={0};Database={1};Uid ={2};Pwd={3};", "127.0.0.1",
"sampledb", "test", "1234!");
string sql = $"Insert Into UserInfo (id,name) values ({id},'{name}')";
using (SqlConnection conn = new SqlConnection(connectString))
{
conn.Open();
SqlCommand cmd = new SqlCommand(sql, conn);
cmd.ExecuteNonQuery();
}
}
//데이터조회
public DataSet GetUserInfo()
{
string connectString = string.Format("Server={0};Database={1};Uid ={2};Pwd={3};", "127.0.0.1",
"sampledb", "test", "1234!");
string sql = "select * from [UserInfo]";
DataSet ds = new DataSet();
using (SqlConnection conn = new SqlConnection(connectString))
{
conn.Open();
SqlDataAdapter da = new SqlDataAdapter(sql, conn);
da.Fill(ds);
}
return ds;
}
}
}
|
2. 데이터저장 및 조회
윈도우 메인프로젝트에서 MssqlLib에서 만들어 놓은 InsertDB와 GetUserInfo함수를 통해 데이터를 저장 및 조회합니다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
|
namespace MssqlTestProject
{
public partial class Form1 : Form
{
MssqlLib mMssqlLib = new MssqlLib();
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
Search();
}
private void Search()
{
DataSet ds = mMssqlLib.GetUserInfo();
dataGridView1.DataSource = ds.Tables[0];
}
private void button2_Click(object sender, EventArgs e)
{
int id = Int32.Parse(txtID.Text);
string name = txtName.Text.Trim();
mMssqlLib.InsertDB(id,name);
Search();
}
}
}
|
실행결과
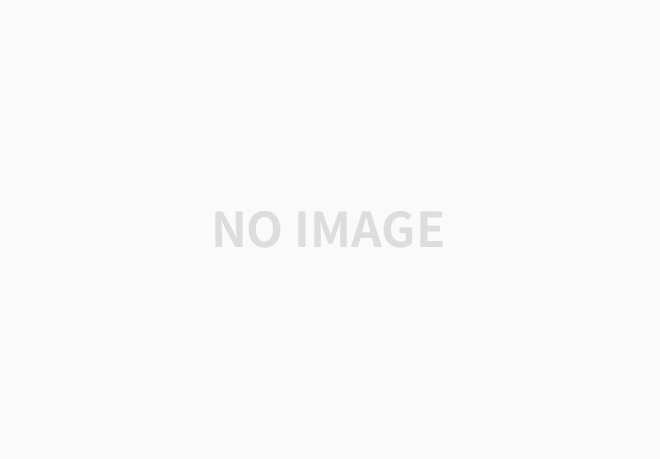
'프로그래밍 > C#' 카테고리의 다른 글
[C#] 0 테이블을 찾을 수 없습니다 (0) | 2022.12.26 |
---|---|
[C#] MSSQL 저장프로시저 사용하기 (0) | 2022.12.26 |
[C#] WebClient 타임아웃 설정 (2) | 2022.12.15 |
[C#] 다국어 처리 방법(MultiLanguage) (0) | 2022.10.28 |
[C#] 서비스프로그램 만들기 (0) | 2022.10.22 |